Eğitimler
StringToInt
ToInt () işlevi, bir String'i bir tamsayı numarasına dönüştürmenizi sağlar.
Bu örnekte, pano bir satırsonu görene kadar bir seri giriş dizesi okur, sonra karakterler basamaksa dizeyi sayıya dönüştürür. Kodu panonuza yükledikten sonra, Arduino IDE seri monitörünü açın, bazı numaralar girin ve gönder tuşuna basın. Yönetim kurulu bu numaraları size tekrar edecektir. Sayısal olmayan bir karakter gönderildiğinde neler olduğunu gözlemleyin.
Gerekli Donanım:
- Arduino veya Genuino Kurulu
Devre
Bu örnek için devre yoktur, ancak kartınızın bilgisayarınıza USB ile bağlanması ve Arduino Yazılımının (IDE) seri monitör penceresinin açık olması gerekir.
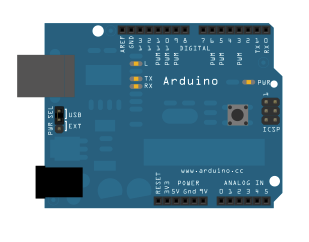
Kod
/*
String to Integer conversion
Reads a serial input string until it sees a newline, then converts the string
to a number if the characters are digits.
The circuit:
- No external components needed.
created 29 Nov 2010
by Tom Igoe
This example code is in the public domain.
http://www.arduino.cc/en/Tutorial/StringToInt
*/
String inString = ""; // string to hold input
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
// send an intro:
Serial.println("\n\nString toInt():");
Serial.println();
}
void loop() {
// Read serial input:
while (Serial.available() > 0) {
int inChar = Serial.read();
if (isDigit(inChar)) {
// convert the incoming byte to a char and add it to the string:
inString += (char)inChar;
}
// if you get a newline, print the string, then the string's value:
if (inChar == '\n') {
Serial.print("Value:");
Serial.println(inString.toInt());
Serial.print("String: ");
Serial.println(inString);
// clear the string for new input:
inString = "";
}
}
}
See Also
- String object- String nesneleri için Referansınız
- CharacterAnalysis- Karşılaştığımız karakter türünü tanımamıza izin veren operatörleri kullanıyoruz.
- StringAdditionOperator- Çeşitli yollarla birlikte dizeler ekleyin.
- StringAppendOperator- Dizelere şeyler eklemek için + = operatörünü ve concat () yöntemini kullanın
- StringCaseChanges- Bir dizenin durumunu değiştirin.
- StringCharacters- Bir dizede belirli bir karakterin değerini alır / ayarlar.
- StringComparisonOperators- Bir dizede belirli bir karakterin değerini alır / ayarlar.
- StringConstructors- Dize nesnelerini başlatın.
- StringIndexOf- Dizede bir karakterin ilk / son örneğini arayın.
- StringLength- Bir dizginin uzunluğunu al.
- StringLengthTrim- Bir dizenin uzunluğunu alın ve kesin.
- StringReplace- Bir dizedeki karakterleri tek tek değiştirin.
- StringStartsWithEndsWith- Belirli bir dizenin hangi karakterlerle / alt dizelerle başladığını veya bittiğini kontrol edin.
- StringSubstring- Belirli bir dize içinde "ifadeler" arayın.