Eğitimler
StringConstructors
String nesnesi , metin dizelerini çeşitli yararlı şekillerde değiştirmenize olanak tanır. Dizelere karakter ekleyebilir, birleştirme yoluyla Dizeleri birleştirebilir, bir Dizenin uzunluğunu alabilir, alt dizeleri arayabilir ve değiştirebilirsiniz ve daha fazlasını yapabilirsiniz. Bu eğitimde String nesnelerini nasıl başlatacağınız gösterilmektedir.
String stringOne = "Hello String"; // using a constant String String stringOne = String('a'); // converting a constant char into a String String stringTwo = String("This is a string"); // converting a constant string into a String object String stringOne = String(stringTwo + " with more"); // concatenating two strings String stringOne = String(13); // using a constant integer String stringOne = String(analogRead(0), DEC); // using an int and a base String stringOne = String(45, HEX); // using an int and a base (hexadecimal) String stringOne = String(255, BIN); // using an int and a base (binary) String stringOne = String(millis(), DEC); // using a long and a base String stringOne = String(5.698, 3); // using a float and the decimal places
Tüm bu yöntemler bir String nesnesi bildirmek için geçerli yollardır. Bunların tümü, String yöntemlerinden herhangi biri kullanılarak işlenebilen bir karakter dizesi içeren bir nesne ile sonuçlanır. Bunları çalışırken görmek için aşağıdaki kodu bir Arduino veya Genuino kartına yükleyin ve Arduino IDE seri monitörünü açın. Her bildirimin sonuçlarını göreceksiniz. Her println () tarafından yazdırılanları üstündeki bildirimle karşılaştırın.
Gerekli Donanım
- Arduino veya Genuino Kurulu
Devre
Bu örnek için devre yoktur, ancak kartınızın bilgisayarınıza USB ile bağlanması ve Arduino Yazılımının (IDE) seri monitör penceresinin açık olması gerekir.
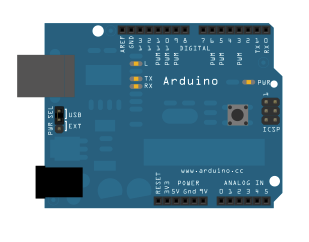
Kod
/*
String constructors
Examples of how to create Strings from other data types
created 27 Jul 2010
modified 30 Aug 2011
by Tom Igoe
This example code is in the public domain.
http://www.arduino.cc/en/Tutorial/StringConstructors
*/
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
// send an intro:
Serial.println("\n\nString Constructors:");
Serial.println();
}
void loop() {
// using a constant String:
String stringOne = "Hello String";
Serial.println(stringOne); // prints "Hello String"
// converting a constant char into a String:
stringOne = String('a');
Serial.println(stringOne); // prints "a"
// converting a constant string into a String object:
String stringTwo = String("This is a string");
Serial.println(stringTwo); // prints "This is a string"
// concatenating two strings:
stringOne = String(stringTwo + " with more");
// prints "This is a string with more":
Serial.println(stringOne);
// using a constant integer:
stringOne = String(13);
Serial.println(stringOne); // prints "13"
// using an int and a base:
stringOne = String(analogRead(A0), DEC);
// prints "453" or whatever the value of analogRead(A0) is
Serial.println(stringOne);
// using an int and a base (hexadecimal):
stringOne = String(45, HEX);
// prints "2d", which is the hexadecimal version of decimal 45:
Serial.println(stringOne);
// using an int and a base (binary)
stringOne = String(255, BIN);
// prints "11111111" which is the binary value of 255
Serial.println(stringOne);
// using a long and a base:
stringOne = String(millis(), DEC);
// prints "123456" or whatever the value of millis() is:
Serial.println(stringOne);
// using a float and the right decimal places:
stringOne = String(5.698, 3);
Serial.println(stringOne);
// using a float and less decimal places to use rounding:
stringOne = String(5.698, 2);
Serial.println(stringOne);
// do nothing while true:
while (true);
}
See Also
- String object- String nesneleri için Referansınız
- CharacterAnalysis- Karşılaştığımız karakter türünü tanımamıza izin veren operatörleri kullanıyoruz.
- StringAdditionOperator- Çeşitli yollarla birlikte dizeler ekleyin.
- StringAppendOperator- Dizelere şeyler eklemek için + = operatörünü ve concat () yöntemini kullanın
- StringCaseChanges- Bir dizenin durumunu değiştirin.
- StringCharacters- Bir dizede belirli bir karakterin değerini alır / ayarlar.
- StringComparisonOperators- Bir dizede belirli bir karakterin değerini alır / ayarlar.
- StringIndexOf- Dizede bir karakterin ilk / son örneğini arayın.
- StringLength- Bir dizginin uzunluğunu al.
- StringLengthTrim- Bir dizenin uzunluğunu alın ve kesin.
- StringReplace- Bir dizedeki karakterleri tek tek değiştirin.
- StringStartsWithEndsWith- Belirli bir dizenin hangi karakterlerle / alt dizelerle başladığını veya bittiğini kontrol edin.
- StringSubstring- Belirli bir dize içinde "ifadeler" arayın.
- StringToInt- Bir String'i tamsayıya dönüştürmenizi sağlar.